ActiveResource
1.ActiveResource
Ruby on Railsで扱うことのできるWebサービスのプロトコールにはSOAPとRESTがあります。SORPはActiveWebServiceパッケージに含まれ、RESTはActiveResourceパッケージに含まれています。Ruby on Rails2.0以降はActiveResourceが標準装備となり、ActiveWebServiceは外出しとなりました。ここでは標準装備されたRESTを扱うこととします。
まず2 つのRailsアプリケーションを作成し、ActiveResourceを使って片方のアプリケーションから別のアプリケーションのリソースにアクセスしデータが授受できることを学びましょう。
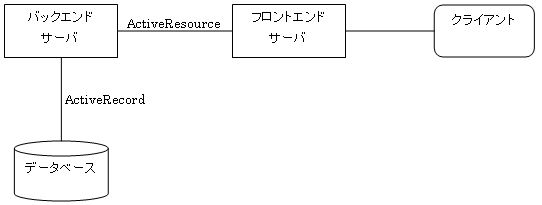
なお、SOAPを扱う場合はgemで簡単に導入できます。
コマンド プロンプト
C:\Ruby>gem install actionwebservice
1-1.ActiveResourceの特徴
ActiveResourceはRuby on Rails 2.0 から追加された機能で、Railsのインストールどきに一緒にインストールされます。Web上のRESTful APIをActiveRecordのモデルと同じようなインターフェースで利用可能にする、いわばObject / RESTful APIマッパーです。とくに同じRailsアプリケーションで提供されるRESTful APIに最適化して設計されていて、Railsの作法に沿って作られたAPIであれば、僅か数行のコーディングで利用できます。その他の特徴は以下のとおりです。
・Rails上ではActiveRecord同様にデータモデルとして扱える。
・ActiveRecord と互換性の高いインターフェース。
・ActiveRecordのValidation エラーを取得可能。
・BASIC 認証を標準サポート。
2.バックエンドとなるアプリケーションの作成
2-1.プロジェクトの生成
(1) プロジェクトAppli012を生成する
(2) 日本語環境の設定
(3) データベースの作成
2-2.テーブル保守プログラムの作成
(1) scaffoldの利用
NetBeansで生成を選択
ジェネレータ(G): scaffold
モデル名(N): Bookmark
属性ペア(フィールド型)(A): title:string url:string comment:text
実行結果exists app/models/
exists app/controllers/
exists app/helpers/
create app/views/bookmarks
exists app/views/layouts/
exists test/functional/
exists test/unit/
create test/unit/helpers/
exists public/stylesheets/
create app/views/bookmarks/index.html.erb
create app/views/bookmarks/show.html.erb
create app/views/bookmarks/new.html.erb
create app/views/bookmarks/edit.html.erb
create app/views/layouts/bookmarks.html.erb
create public/stylesheets/scaffold.css
create app/controllers/bookmarks_controller.rb
create test/functional/bookmarks_controller_test.rb
create app/helpers/bookmarks_helper.rb
create test/unit/helpers/bookmarks_helper_test.rb
route map.resources :bookmarks
dependency model
exists app/models/
exists test/unit/
exists test/fixtures/
create app/models/bookmark.rb
create test/unit/bookmark_test.rb
create test/fixtures/bookmarks.yml
create db/migrate
create db/migrate/20100210045330_create_bookmarks.rb
(2) マイグレーションの実行
NetBeansで[データベースマイグレーション]→[現在のバージョンへ]を選択します。
実行結果(in D:/Rails_Projects/Appli012)
== CreateBookmarks: migrating ================================================
-- create_table(:bookmarks)
-> 0.1250s
== CreateBookmarks: migrated (0.1250s) =======================================
3.フロントエンドとなるアプリケーションの作成
3-1.プロジェクトの生成
(1) プロジェクトAppli014を生成する
(2) 日本語環境の設定
(3) データベースの作成
3-2.テーブル保守アプリケーションの作成
(1) scaffoldの利用
NetBeansで[生成..]を選択
ジェネレータ(G): scaffold
モデル名(N): Bookmark
属性ペア(フィールド型)(A): title:string url:string comment:text
実行結果exists app/models/
exists app/controllers/
exists app/helpers/
create app/views/bookmarks
exists app/views/layouts/
exists test/functional/
exists test/unit/
create test/unit/helpers/
exists public/stylesheets/
create app/views/bookmarks/index.html.erb
create app/views/bookmarks/show.html.erb
create app/views/bookmarks/new.html.erb
create app/views/bookmarks/edit.html.erb
create app/views/layouts/bookmarks.html.erb
create public/stylesheets/scaffold.css
create app/controllers/bookmarks_controller.rb
create test/functional/bookmarks_controller_test.rb
create app/helpers/bookmarks_helper.rb
create test/unit/helpers/bookmarks_helper_test.rb
route map.resources :bookmarks
dependency model
exists app/models/
exists test/unit/
exists test/fixtures/
create app/models/bookmark.rb
create test/unit/bookmark_test.rb
create test/fixtures/bookmarks.yml
create db/migrate
create db/migrate/20100210051742_create_bookmarks.rb
(2) マイグレーションファイルの削除
frontendではDB は使わないので削除しておきます。
(3) モデルクラスの書き換え
基底クラスをActiveResource::Baseにします。これによって Bookmark クラスはActiveResource のモデルになり、 DB の代わりに self.site で指定されたサイトの Web API にアクセスして、データの取得・保存を行います。
/app/controllers/bookmark_controller.rbclass Bookmark < ActiveResource::Base
self.site = 'http://127.0.0.1:3000/'
self.user = 'root'
self.password = 'pwMySQL'alias_method :new_record?, :new?
def initialize(attr={})
super({:title => nil, :url => nil, :comment => nil}.update(attr))
end
end
(4) コントローラの修正
/app/models/bookmark.rbclass BookmarksController < ApplicationController
# GET /bookmarks
# GET /bookmarks.xml
def index
@bookmarks = Bookmark.find(:all)respond_to do |format|
format.html # index.html.erb
format.xml { render :xml => @bookmarks }
end
end
:
:
# GET /bookmarks/1/edit
def edit
@bookmark = Bookmark.find(params[:id])
end
:
:
# PUT /bookmarks/1
# PUT /bookmarks/1.xml
def update
@bookmark = Bookmark.find(params[:id])respond_to do |format|
@bookmark.title = params[:bookmark][:title]
@bookmark.url = params[:bookmark][:url]
@bookmark.comment = params[:bookmark][:comment]
if @bookmark.save
flash[:notice] = 'Bookmark was successfully updated.'
format.html { redirect_to(@bookmark) }
format.xml { head :ok }
else
format.html { render :action => "edit" }
format.xml { render :xml => @bookmark.errors, :status => :unprocessable_entity }
end
end
end
:
:
end
(5) 背景色の変更
Appli012とAppli014を区別するため、表示画面の背景色を「白」から「青系」に変更します。
/public/stylesheets/scaffold.cssbody { background-color: #8888ff; color: #333; }body, p, ol, ul, td {
font-family: verdana, arial, helvetica, sans-serif;
font-size: 13px;
line-height: 18px;
}pre {
background-color: #eee;
padding: 10px;
font-size: 11px;
}a { color: #000; }
a:visited { color: #666; }
a:hover { color: #fff; background-color:#000; }.fieldWithErrors {
padding: 2px;
background-color: red;
display: table;
}#errorExplanation {
width: 400px;
border: 2px solid red;
padding: 7px;
padding-bottom: 12px;
margin-bottom: 20px;
background-color: #f0f0f0;
}#errorExplanation h2 {
text-align: left;
font-weight: bold;
padding: 5px 5px 5px 15px;
font-size: 12px;
margin: -7px;
background-color: #c00;
color: #fff;
}#errorExplanation p {
color: #333;
margin-bottom: 0;
padding: 5px;
}#errorExplanation ul li {
font-size: 12px;
list-style: square;
}
4.動作確認
(1) Appli012(Backendアプリケーション)の起動
NetBeansで[実行]を選択する
port番号3000で起動されます。
(2) Appli014(Frontendアプリケーション)の起動
port番号3001で起動されます。
(3) backendアプリケーションの実行
クライアントからhttp://127,0,0,1:3000/bookmarks/を入力してbackendアプリケーションを実行します。
(4) frontendアプリケーションの実行
クライアントからhttp://127,0,0,1:3001/bookmarks/を入力してfrontendアプリケーションを実行します。